Implementing Stream with lambdas
Posted on January 1, 2020
Tags: functional
import numpy as np
import matplotlib.pyplot as plt
1 Plot a recurrence relation
\[ S_{t+1} = (1+r)\times S_t \]
1.1 Naive (loop method)
= 0.025 # interest rate
r = 50 # end date
T = np.empty(T+1) # an empty NumPy array, to store all b_t
b 0] = 10 # initial balance
b[
for t in range(T):
+1] = (1 + r) * b[t]
b[t
='bank balance')
plt.plot(b, label
plt.plot(xdata,ydata) plt.show()
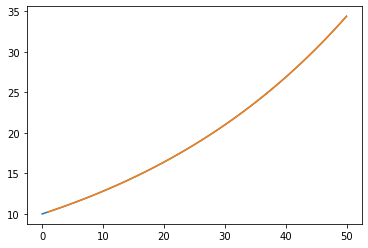
1.2 Functional zipWith approach
\[[id, f, f\circ f, f\circ f\circ f .. f^n ]\] \[ apply\ to\] \[[10,10,10,10...]\]
funcPow
=\(f^n\)zipWith
applys elementwise each function in the function-list with it’s respective argument in the argument list.
= 0.025
r = lambda x: (1+r)*x
S = [i for i in range(1,51)]
xdata
def funcPow(n,f,x):
if n == 0:
return x
else:
return f(funcPow(n-1,f,x))
def zipWith(a,b):
= zip(a,b)
c return [(x[0])(x[1]) for x in c]
= list(map(lambda n : (lambda x: funcPow(n,S,x)),xdata))
applylist = [10]*50
ones = [applylist[i] for i in range(0,50)]
flist
= zipWith(flist,ones)
output plt.plot(zipWith(flist,ones))
[<matplotlib.lines.Line2D at 0x7fc9291d4ca0>]
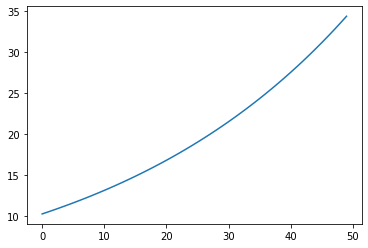
1.3 LazyList method
\[[x, f(x), (f\circ f)(x), (f\circ f\circ f)(x), .. f^n(x)..f^\infty(x) ]\]
- lazylist needs a function and a base element which is 10
= 0.025
r = lambda x: (1+r)*x
S class StreamLinkedList:
def __init__(self,f,data):
self.data = data
self.next = lambda : StreamLinkedList(f,f(data))
def __repr__(self):
return str(self.data)
def take(n,xs):
if n == 1:
return [xs.data]
else:
= take(n-1,xs.next()) #take returns a list
IH return [xs.data]+IH
= StreamLinkedList(S,10)
a
=take(50,a)
gg plt.plot(xdata,gg)
[<matplotlib.lines.Line2D at 0x7fc93c1b2d00>]
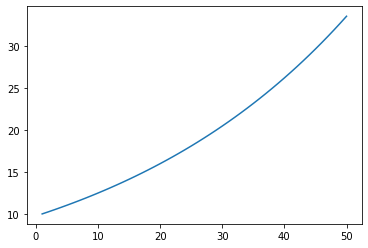