3d plots and Contour plot
Posted on October 15, 2021
Tags: codeetc
ax.contour3D
specifically requires theax
setup.plt.contour
plt.contourf
allows immediately plottingnp.meshgrid(x,y) = X,Y
where X,Y are broadcasts of x and y to the same dimension- input x.shape is (2,1)
- input y shape is (3,1)
- rows of X are broadcasted copies of x
- X.shape is (2,3)
- cols of Y are broadcasted copies of y
- Y.shape is (2,3)
- Y.shape is (2,3)
- Notice that elementwise product of X and Y is the product space of x and y.
In other words, it is all combinations of x and y.
X,Y = meshgrid(x=[1,2],y=[3,4,5])
\[ x=\begin{bmatrix} {\color{red}1} & {\color{red}2} \end{bmatrix} \qquad y=\begin{bmatrix} {\color{blue}3} & {\color{blue}4} &{\color{blue}5} \end{bmatrix} \qquad X = \begin{bmatrix} {\color{red}1} & {\color{red}2} \\ 1 & 2 \\ 1 & 2 \end{bmatrix} \qquad Y = \begin{bmatrix} {\color{blue}3} & 3 \\ {\color{blue}4} & 4 \\ {\color{blue}5} & 5 \end{bmatrix}\]
\[X \otimes Y = \begin{bmatrix} (1,3) & (2,3) \\ (1,4) & (2,4) \\ (1,5) & (2,5) \end{bmatrix}\]
from mpl_toolkits import mplot3d
import numpy as np
import matplotlib.pyplot as plt
def cont3d(X,Y,Z,plottype="wireframe"):
= plt.figure()
fig = plt.axes(projection='3d')
ax if plottype == "contour3D":
50, cmap='viridis')
ax.contour3D(X,Y,Z, elif plottype == "surface":
="coolwarm", linewidth=0, antialiased=False)
ax.plot_surface(X, Y, Z, cmapelif plottype == "wireframe":
=5, cstride=5)
ax.plot_wireframe(X, Y, Z, rstride
'x')
ax.set_xlabel('y')
ax.set_ylabel('z')
ax.set_zlabel(# ax.set_title('3D contour')
return (plt,ax)
def f(x, y):
return np.sqrt(x ** 2 + y ** 2)
= np.linspace(-6, 6, 30)
x = np.linspace(-6, 6, 30)
y
= np.meshgrid(x, y)
X, Y = f(X, Y)
Z print(X.shape,Y.shape)
= cont3d(X,Y,Z,plottype="contour3D")
fig,ax
fig.show()= cont3d(X,Y,Z,plottype="surface")
fig,ax
fig.show()= cont3d(X,Y,Z,plottype="wireframe")
fig,ax fig.show()
(30,)
(30, 30) (30, 30)
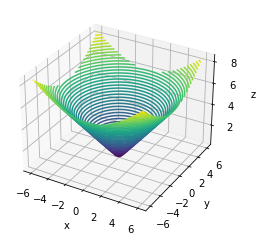
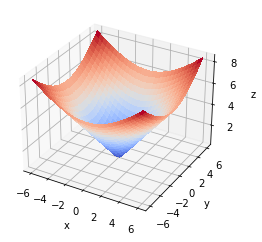
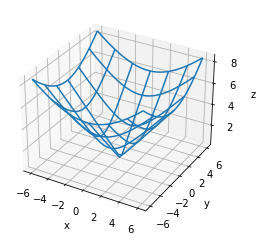
1 Simple Contour plots
- In 2d contour plots, color is the z-axis.
plt.contourf(X,Y,Z)
<matplotlib.contour.QuadContourSet at 0x7fc419419910>
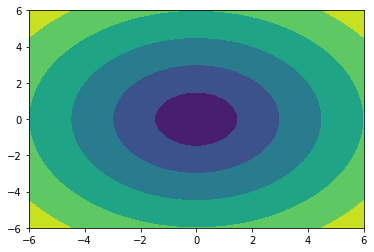
plt.contour(X,Y,Z)
<matplotlib.contour.QuadContourSet at 0x7fc4192cc670>
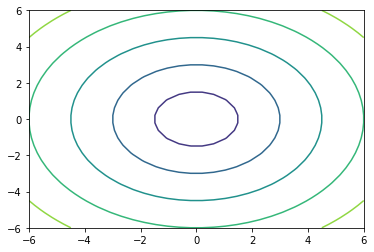
2 3d Plot
2.1 Plot random
def f(x, y):
return np.random.poisson(size=x.shape)
= np.linspace(-6, 6, 30)
x = np.linspace(-6, 6, 30)
y
= np.meshgrid(x, y)
X, Y = f(X, Y)
Z
= cont3d(X,Y,Z,plottype="surface")
fig,ax fig.show()
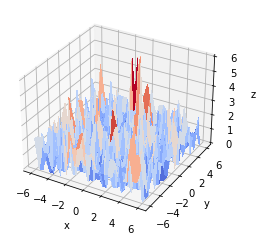
def f(x, y):
return np.random.rand(*x.shape)
= np.linspace(-6, 6, 30)
x = np.linspace(-6, 6, 30)
y
= np.meshgrid(x, y)
X, Y = f(X, Y)
Z
= cont3d(X,Y,Z,plottype="contour3D")
fig,ax fig.show()
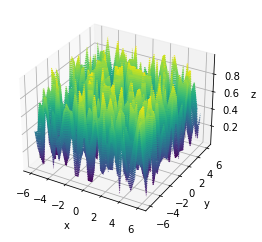
def f(x, y):
return y
= np.linspace(-6, 6, 30)
x = np.linspace(-6, 6, 30)
y
= np.meshgrid(x, y)
X, Y = f(X, Y)
Z
0].show() cont3d(X,Y,Z)[
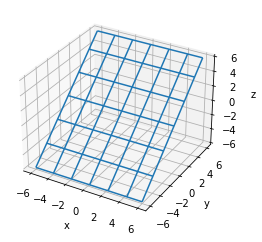
2.2 Manual Tuning Neural Net
x1 | x2 | LinearFunc | Sigmoid | Actual x1 OR x2 |
---|---|---|---|---|
0 | 0 | (0)(10)+(0)(10)+(-5)= -5 | σ(-5)=0.00669≅0 | 0 |
0 | 1 | (0)(10)+(1)(10)+(-5)= 5 | σ(5)=0.99330≅1 | 1 |
1 | 0 | (1)(10)+(0)(10)+(-5)= 5 | σ(5)=0.99330≅1 | 1 |
1 | 1 | (1)(10)+(1)(10)+(-5)= 15 | σ(15)=0.99999≅1 | 1 |
# Defina a collection of inputs to test OR and AND
= np.array([
my_x_collection 0, 0],
[0, 1],
[1, 0],
[1, 1],
[ ])
def LinearFunction(x1,x2,w1,w2,b):
return (x1*w1 + x2*w2) + b
def Sigmoid_2d(x1, x2, w1, w2, b):
return 1/(1+np.exp(-LinearFunction(x1,x2,w1,w2,b)))
- These are the weights we discovered, w1=10,w2=10,b=-5
= 10
w1 = 10
w2 = -5 b
print("Input\tLinear\tSigmoid")
for x in my_x_collection:
= x[0]
x1 = x[1]
x2 = LinearFunction(x1,x2,w1,w2,b)
LinearOutput = Sigmoid_2d(x1,x2,w1,w2,b)
SigmoidOutput print(f"{x}\t{LinearOutput}\t{SigmoidOutput}")
Input Linear Sigmoid
[0 0] -5 0.0066928509242848554
[0 1] 5 0.9933071490757153
[1 0] 5 0.9933071490757153
[1 1] 15 0.999999694097773
= np.linspace(-1, 1, 30)
x = np.linspace(-1, 1, 30)
y
= np.meshgrid(x, y)
X, Y = Sigmoid_2d(X, Y, w1, w2, b)
Z
= cont3d(X,Y,Z)
fig,ax 0,0,0)
ax.scatter(0,1,1)
ax.scatter(1,0,1)
ax.scatter(1,1,1)
ax.scatter( fig.show()
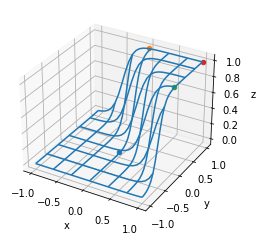